Part 3: Approval UI
Background: LangGraph implementation details
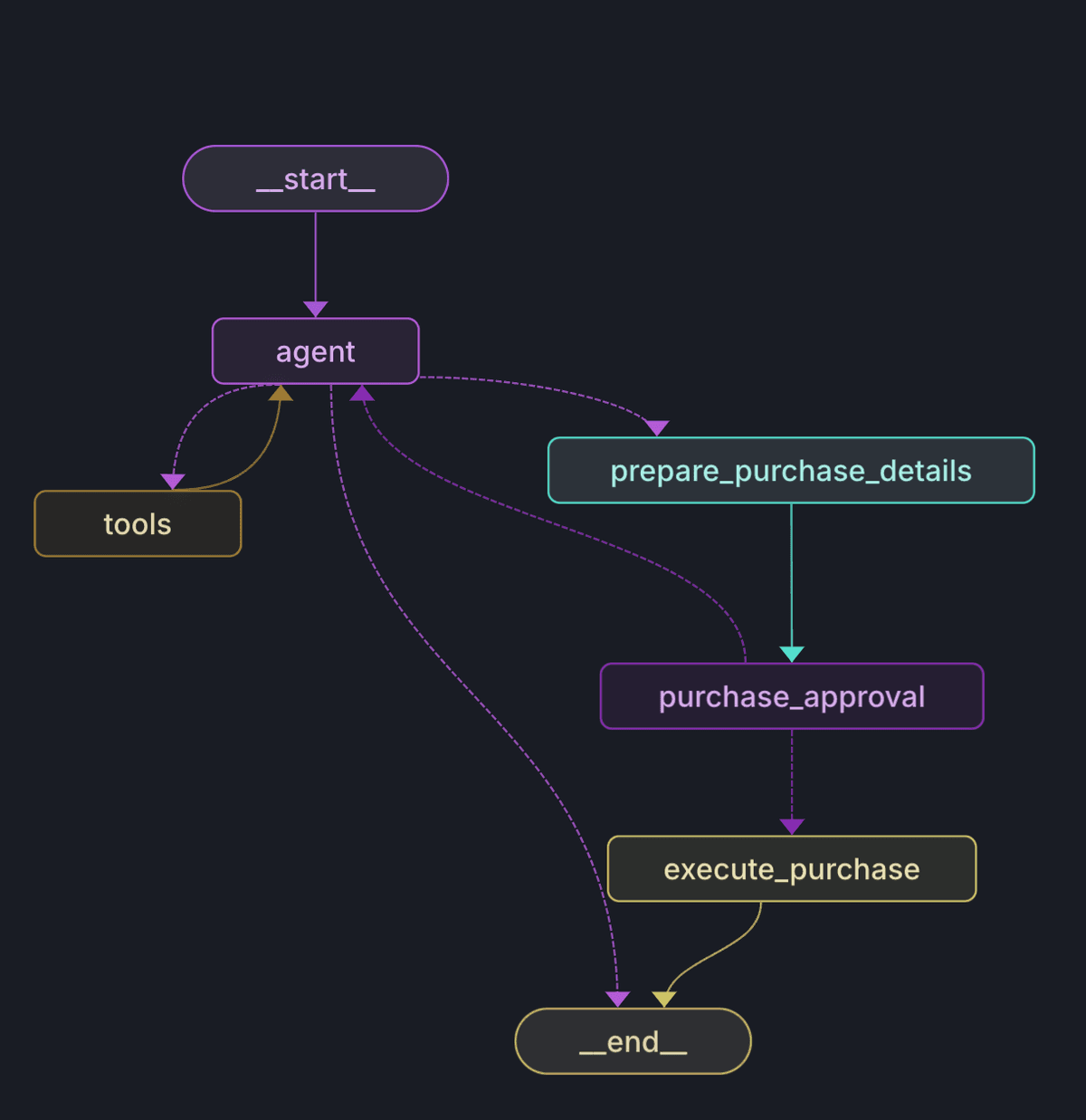
Our LangGraph backend interrupts the purchase_stock
tool execution in order to ensure the user confirms the purchase. The user confirms the purchase by submitting a tool message with the approve
field set to true
.
Add approval UI
We create a new file under /components/tools/purchase-stock/PurchaseStockTool.tsx
to define the tool.
First, we define the tool arguments and result types:
Then we use makeAssistantToolUI
to define the tool UI:
Finally, we add a TransactionConfirmationPending
component to ask for approval.
This requires shadcn/ui's Card
and Button
components. We will install them as a dependency.
Then create a new file under /components/tools/purchase-stock/transaction-confirmation-pending.tsx
to define the approval UI.
Bind approval UI
Try it out!
Ask the assistant to buy 5 shares of Tesla. You should see the following appear:
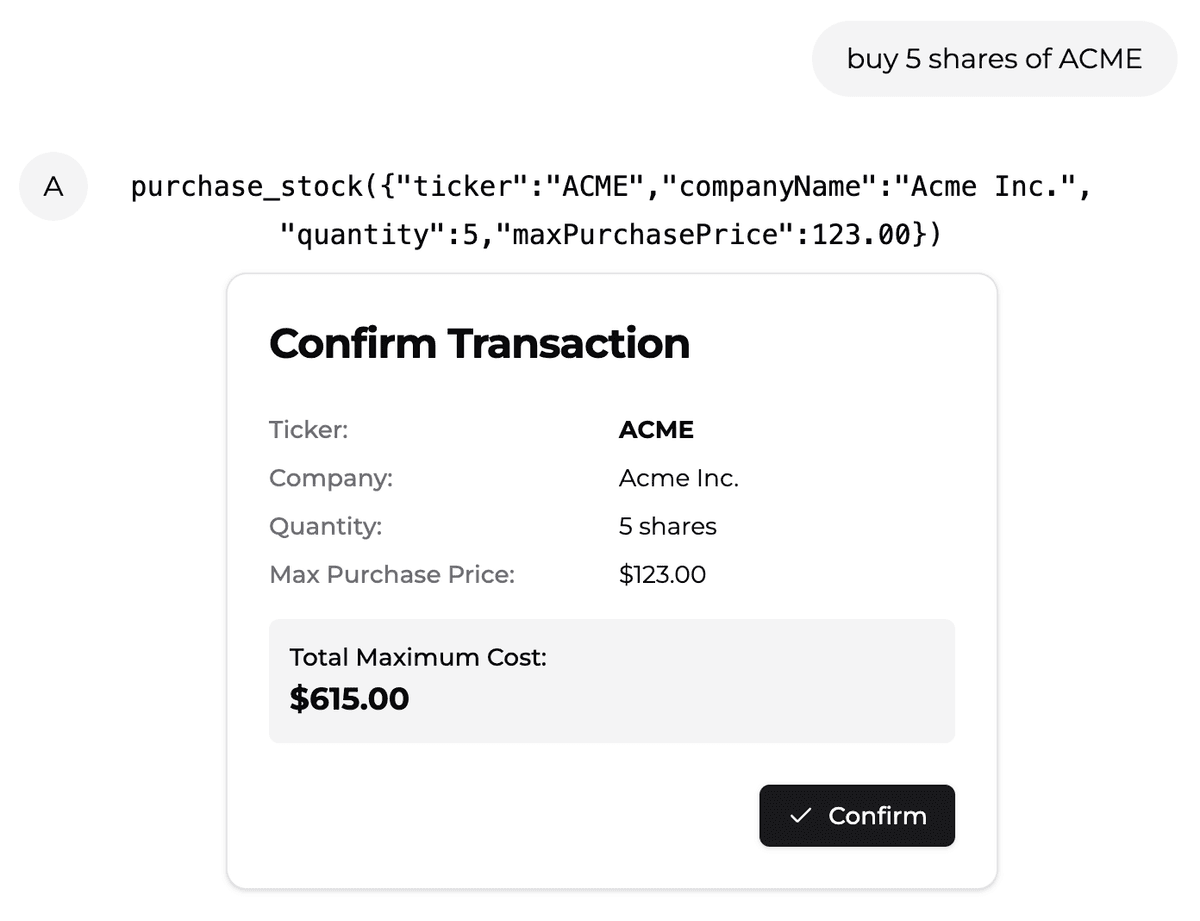
Add TransactionConfirmationFinal
to show approval result
We will add a component to display the approval result.
Update PurchaseStockTool
We will import the new <TransactionConfirmationFinal />
component and use it in the render
function whenever an approval result is available.
Try it out!
Confirm the purchase of shares. You should see the approval confimration UI appear.
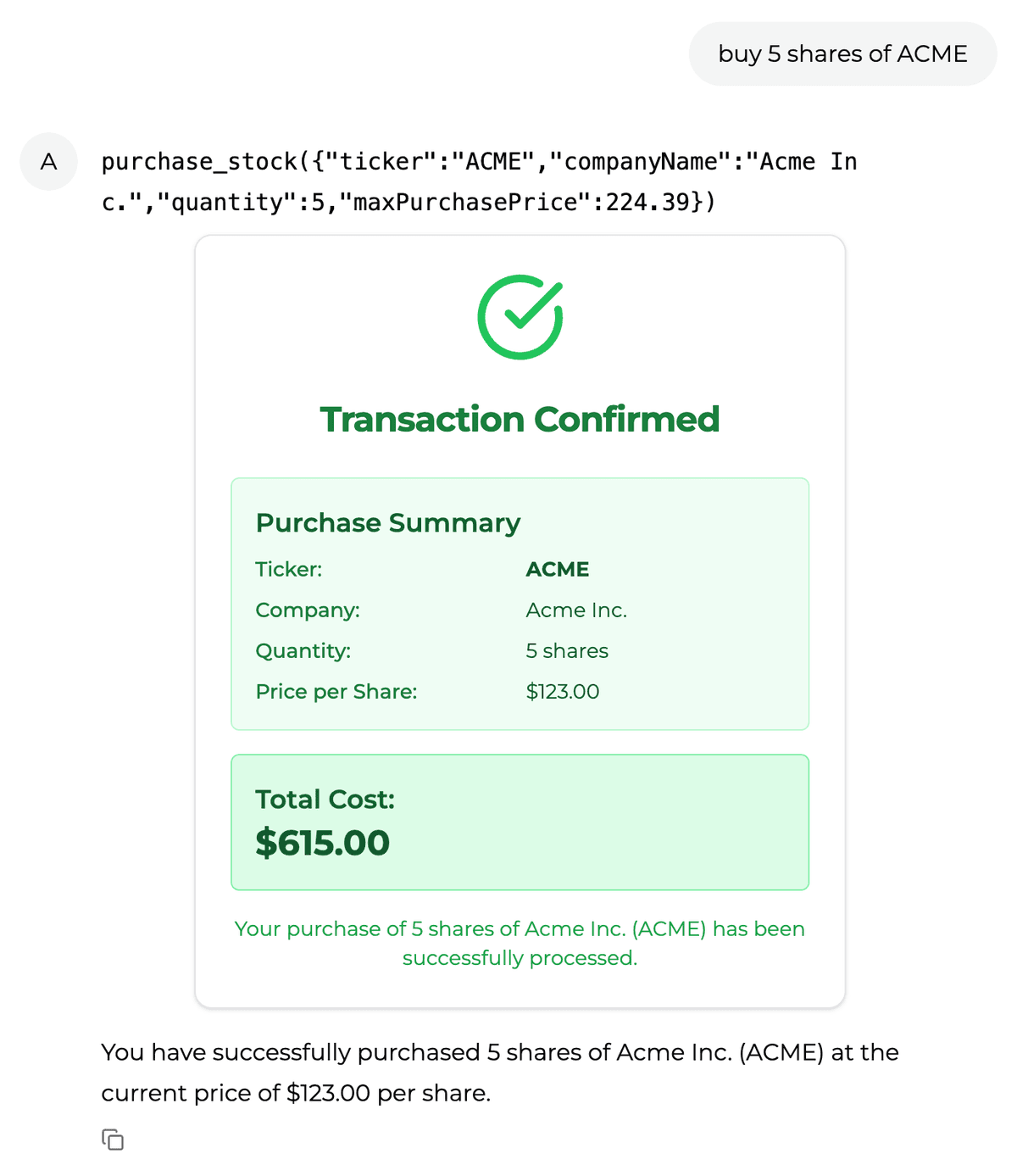